Contents
- 0.1 Brief overview of PowerShell
- 0.2 Importance of string manipulation in PowerShell
- 0.3 Introduction to concatenating strings in PowerShell
- 0.4 Scope of the blog: best practices and techniques for concatenating strings in PowerShell
- 1 Basic String Concatenation in PowerShell
- 2 Using the Join Operator
- 3 Using the Format (-f) Operator
- 4 Concatenating Strings and Integers
- 5 Concatenating Strings with Variables
- 6 Advanced Concatenation Techniques
- 7 Other Commonly Used String Functions in PowerShell
- 8 Best Practices for Concatenating Strings in PowerShell
- 9 Conclusion
- 10 Discover More Windows Tips
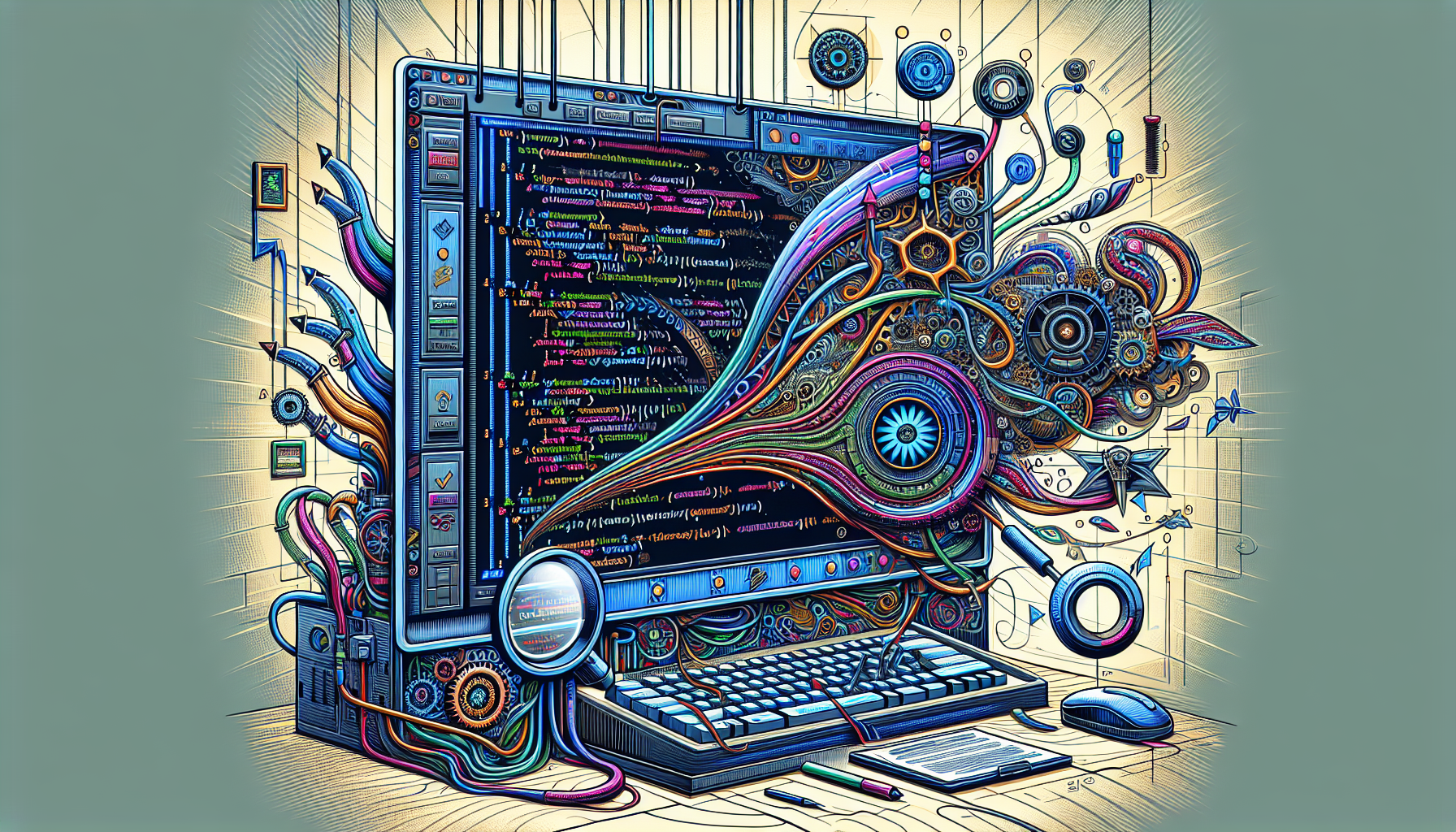
Brief overview of PowerShell
PowerShell is a versatile scripting language and automation framework developed by Microsoft, enabling administrators to automate repetitive tasks and manage systems more efficiently.
Importance of string manipulation in PowerShell
String manipulation is crucial in PowerShell, as it allows users to extract, modify, and create data, thus streamlining tasks and enhancing script readability and functionality.
Introduction to concatenating strings in PowerShell
Concatenating strings is a fundamental aspect of string manipulation, merging two or more strings to create a new, combined string, often used for reporting, logging, and data presentation.
Scope of the blog: best practices and techniques for concatenating strings in PowerShell
This blog delves into the best practices and techniques for concatenating strings in PowerShell, providing insights for efficient, readable, and performant string concatenation.
Basic String Concatenation in PowerShell
When working with PowerShell, you’ll often need to manipulate strings, and one of the most basic operations is string concatenation. In this section, we’ll explore the simplest ways to concatenate strings in PowerShell, such as using the plus (+) operator and concatenating strings and variables directly. We’ll also provide examples to help you better understand these techniques.
Concatenating Strings Using the Plus (+) Operator
One of the most straightforward ways to concatenate strings in PowerShell is by using the plus (+) operator. This method allows you to join two or more strings together simply by adding them. For example, you can concatenate the strings “Hello” and “World” as follows:
$greeting = "Hello" + " " + "World"
Write-Host $greeting
This code snippet will output “Hello World”. It’s important to note that the plus (+) operator requires a space character between the strings to ensure proper spacing in the final output.
Concatenating Strings and Variables Directly
You can also concatenate strings and variables directly in PowerShell without using any operator. This technique is useful when you need to combine a string with a variable value. For example, consider the following code snippet:
$name = "John"
$greeting = "Hello, $name!"
Write-Host $greeting
This code will output “Hello, John!”. Notice that the variable $name is included directly in the string without any additional operator. PowerShell automatically replaces the variable with its value during string concatenation.
Example Scenarios and Their Solutions
Let’s consider a few example scenarios where you might need to concatenate strings in PowerShell:
- Combining file paths: You may need to concatenate strings to create a file path. For example:
$folder = "C:\Users\John\Documents"
$filename = "file.txt"
$filepath = $folder + "\" + $filename
Write-Host $filepath
This code will output “C:\Users\John\Documents\file.txt”.
- Generating a custom message: You might need to generate a custom message based on user input or variable values. For example:
$weather = "sunny"
$message = "Today's weather is " + $weather + ". Have a great day!"
Write-Host $message
This code will output “Today’s weather is sunny. Have a great day!”.
Understanding these basic string concatenation techniques in PowerShell will help you create more powerful scripts and improve your overall scripting skills. In the following sections, we’ll explore more advanced concatenation methods and best practices to further enhance your PowerShell proficiency.
Using the Join Operator
When it comes to concatenating strings in PowerShell, the join operator offers an efficient and versatile alternative to the plus (+) operator. This section will outline the benefits of using the join operator, its syntax and usage, and provide example scenarios to demonstrate its effectiveness.
Benefits of Using the Join Operator for Concatenation
One of the key advantages of using the join operator is its ability to concatenate multiple strings or string arrays in a single command. This can lead to improved readability and reduced complexity in your scripts. Additionally, the join operator allows you to specify a delimiter between the concatenated strings, making it easier to format your output as needed.
Syntax and Usage of the Join Operator
The syntax for using the join operator in PowerShell is as follows:
-join (array of strings)
Alternatively, you can use the join operator with a specified delimiter:
delimiter -join (array of strings)
Here, the ‘delimiter’ can be any character or sequence of characters that you want to insert between the concatenated strings.
Example Scenarios and Their Solutions
Scenario 1: Concatenating an array of strings without a delimiter
$names = @("John", "Jane", "Doe")
$concatenatedNames = -join $names
Write-Host $concatenatedNames
The output will be:
JohnJaneDoe
Scenario 2: Concatenating an array of strings with a delimiter
$names = @("John", "Jane", "Doe")
$concatenatedNames = "," -join $names
Write-Host $concatenatedNames
The output will be:
John,Jane,Doe
As demonstrated in the examples, the join operator provides a simple yet powerful way to concatenate strings in PowerShell while maintaining script readability and allowing for easy customization of the output format.
Using the Format (-f) Operator
When it comes to concatenating strings in PowerShell, the format (-f) operator offers several advantages over the traditional plus (+) operator. This operator allows for a more readable and efficient way to combine strings while providing a consistent syntax for various scenarios. In this section, we’ll discuss the benefits of using the format operator for concatenation, its syntax and usage, and share some practical examples.
Advantages of Using the Format Operator for Concatenation
One of the primary benefits of using the format operator is improved readability. With the format operator, it’s easier to visualize the final output of the concatenated string. Additionally, this operator simplifies the process of concatenating multiple strings and variables, making it a more efficient method for complex concatenation tasks.
Syntax and Usage of the Format Operator
The syntax for the format operator is straightforward, using the -f operator followed by curly braces {}. Inside the curly braces, you can place placeholders, represented by numbers, that correspond to the position of the values to be inserted. Here’s a simple example:
$template = "Hello, {0}! Welcome to {1}."
$result = $template -f "John", "PowerShell"
In this example, the format operator replaces the placeholders {0} and {1} with the corresponding values “John” and “PowerShell.” The result will be the string “Hello, John! Welcome to PowerShell.”
Example Scenarios and Their Solutions
Let’s explore a few scenarios where using the format operator can help improve the readability and efficiency of your string concatenation tasks:
- Concatenating multiple strings and variables: The format operator is especially useful when you need to combine several strings and variables. For example, you may want to create a log entry that includes a timestamp, username, and action. With the format operator, you can easily create a template string and insert the required values:
$logTemplate = "{0} - User {1} performed action: {2}"
$logEntry = $logTemplate -f (Get-Date), $username, $action
- Formatting numbers: The format operator can also help with formatting numbers in your strings. For example, you may want to display a percentage with two decimal places. The format operator allows you to specify the number format for each placeholder:
$percentTemplate = "The progress is {0:N2}%"
$progress = $percentTemplate -f $percentage
- Escaping curly braces: If you need to include curly braces in your concatenated string, the format operator can help you escape them properly. To include a literal curly brace, simply double it up in your template string:
$codeTemplate = "The PowerShell command is: {{ Get-Item {0} }}"
$command = $codeTemplate -f
$path
In conclusion, the format operator is a powerful tool for concatenating strings in PowerShell. By providing improved readability, efficiency, and consistency in syntax, the format operator is an essential technique for anyone working with PowerShell scripts.
Concatenating Strings and Integers
When working with PowerShell, you may encounter situations where you need to concatenate strings and integers. This can present some challenges, as PowerShell treats strings and integers as different data types. In this section, we will explore the challenges faced while concatenating strings and integers and discuss techniques for overcoming these challenges, along with example scenarios and their solutions.
Challenges Faced While Concatenating Strings and Integers
Directly concatenating strings and integers can result in unexpected behavior and errors. For instance, when using the plus (+) operator, PowerShell may attempt to perform arithmetic addition instead of concatenation. This is because the plus (+) operator is also used for addition, and PowerShell tries to add the integer to the string, which is not valid. Additionally, directly concatenating strings and integers can lead to code that is difficult to read and maintain.
Techniques for Concatenating Strings and Integers in PowerShell
There are several techniques you can use to concatenate strings and integers in PowerShell, ensuring that the operation is performed correctly and the code remains readable:
- Convert the integer to a string using the ToString() method. This allows you to concatenate the resulting string with another string using the plus (+) operator.
- Use the -f format operator to specify how the integer should be inserted into the string. This method is particularly useful when you need to format the integer in a specific way, such as adding leading zeros or controlling the number of decimal places.
- Use the string interpolation feature in PowerShell. With string interpolation, you can embed expressions, including integers, directly into a string. PowerShell will automatically evaluate the expressions and convert the results to strings.
Example Scenarios and Their Solutions
Let’s take a look at some example scenarios and demonstrate how to use the techniques discussed above to concatenate strings and integers in PowerShell:
- Scenario: You want to display the message “The total number of files is 10” by concatenating a string and an integer.
Solution: Use the ToString() method to convert the integer to a string and concatenate it with the other string:$integer = 10
$message = "The total number of files is " + $integer.ToString()
- Scenario: You want to display a date in the format “2021-09-01” by concatenating strings and integers.
Solution: Use the -f format operator to insert the integers into the string:$year = 2021
$month = 9
$day = 1
$date = "{0}-{1:D2}-{2:D2}" -f $year, $month, $day
- Scenario: You want to display the message “The price is $9.99” by concatenating a string and a float.
Solution: Use string interpolation to embed the float directly into the string:$price = 9.99
$message = "The price is $($price)"
By mastering these techniques for concatenating strings and integers in PowerShell, you can overcome the challenges associated with combining different data types and write more effective and readable scripts.
Concatenating Strings with Variables
Concatenating strings with variables is an essential skill in PowerShell scripts, as it allows for dynamic and customizable output. In this section, we will explore the importance of concatenating strings with variables and examine various techniques and examples.
Importance of Concatenating Strings with Variables in PowerShell Scripts
PowerShell scripts often involve combining static text with dynamic data from variables, making the ability to concatenate strings with variables crucial for effective scripting. This skill allows for increased flexibility and adaptability in your scripts, creating outputs tailored to specific situations or user inputs. In addition, properly concatenating strings with variables can make your code more readable, maintainable, and efficient.
Techniques for Concatenating Strings with Variables
There are several techniques for concatenating strings with variables in PowerShell, each with its own benefits and use cases. These methods include:
- Direct concatenation using the plus (+) operator
- Using the join operator
- Utilizing the format (-f) operator
- Employing the Concat() method
Choosing the appropriate technique for your specific scenario will depend on factors such as the complexity of the script, the types of data being concatenated, and personal preferences.
Example Scenarios and Their Solutions
Let’s look at some example scenarios where concatenating strings with variables is necessary and explore the solutions using the various techniques mentioned above.
Scenario 1: Concatenating a user’s name and age to create a personalized greeting.
$name = "John"
$age = 30
$greeting = "Hello, " + $name + "! You are " + $age + " years old."
Write-Output $greeting
Scenario 2: Generating a report title by concatenating a date and a static string.
$date = Get-Date -Format "yyyy-MM-dd"
$title = "Sales Report - " + $date
Write-Output $title
Scenario 3: Creating a file path by combining a folder path, file name, and file extension stored in variables.
$folderPath = "C:\Users\John\Documents"
$fileName = "Report"$fileExtension = ".txt"
$filePath = $folderPath + "\" + $fileName + $fileExtension
Write-Output $filePath
By mastering the techniques discussed in this section and understanding the importance of concatenating strings with variables, you can create more dynamic and effective PowerShell scripts.
Advanced Concatenation Techniques
In addition to basic concatenation techniques, PowerShell offers advanced methods for more complex scenarios. These methods can enhance performance, readability, and maintainability of your scripts. In this section, we will discuss two advanced concatenation techniques: the Concat() method and the StringBuilder class.
Using the Concat() method
The Concat() method is a powerful and efficient way to concatenate strings in PowerShell. It belongs to the System.String class and accepts multiple strings as arguments. The method returns a new string that is a combination of all the input strings. This method is particularly useful when concatenating a large number of strings, as it eliminates the need for multiple concatenation operations.
Here’s an example of using the Concat() method:
$firstName = "John"
$lastName = "Doe"
$age = 30
$result = [System.String]::Concat($firstName, " ", $lastName, " is ", $age, " years old.")
Write-Host $result
This script will output: “John Doe is 30 years old.”
Using the StringBuilder class
The System.Text.StringBuilder class is another advanced method for concatenating strings in PowerShell. It offers an efficient and flexible way to build and manipulate strings, especially when dealing with large strings or repeated concatenation operations. The StringBuilder class is designed to minimize the performance impact of string manipulation, as it avoids creating a new string object for each concatenation.
Here’s an example of using the StringBuilder class:
$stringBuilder = New-Object System.Text.StringBuilder
$stringBuilder.Append("John")$stringBuilder.Append(" ")$stringBuilder.Append("Doe")$stringBuilder.Append(" is ")$stringBuilder.Append(30)$stringBuilder.Append(" years old.")
$result = $stringBuilder.ToString()Write-Host $result
This script will output: “John Doe is 30 years old.”
Example Scenarios and Their Solutions
Let’s explore some real-world scenarios where advanced concatenation techniques can be useful:
Scenario 1: You need to generate a large CSV file with thousands of rows. Using basic concatenation techniques may result in slow performance and high memory usage. In this case, the StringBuilder class can significantly improve performance by minimizing the number of string objects created during the concatenation process.
Scenario 2: You have to concatenate multiple strings with different data types, such as integers, floats, and dates. Using the Concat() method or the StringBuilder class can simplify this process and make your code more readable by handling the conversion of data types automatically.
In conclusion, mastering advanced concatenation techniques in PowerShell can help you write more efficient, flexible, and maintainable scripts. These techniques are especially useful when working with large strings or complex data types, enabling you to tackle challenging string manipulation tasks with ease.
Other Commonly Used String Functions in PowerShell
While concatenating strings in PowerShell is essential, there are other string functions that can significantly enhance your scripting capabilities. Two of the most commonly used functions are the Split() function and the Replace function. This section will delve into the usage and examples of each function, providing you with a comprehensive understanding of their applications.
Split() Function
The Split() function is a versatile tool that allows you to break a string into smaller parts or elements, based on a specified delimiter. This function is particularly useful when working with data that contains multiple values separated by a common character, such as CSV files or log files. The syntax for using the Split() function is as follows:
$String.Split('Delimiter')
For example, if you have a string containing a list of names separated by commas, you can use the Split() function to create an array of individual names:
$Names = "Alice,Bob,Charlie"
$NameArray = $Names.Split(',')
Replace Function
The Replace function enables you to substitute a specific substring or pattern within a string with another string. This function is useful for modifying the contents of a string, such as replacing outdated information, correcting errors, or changing the format of data. The syntax for using the Replace function is:
$String.Replace('OldString', 'NewString')
For instance, if you have a string containing an incorrect date format and you wish to change it to the correct format, you can use the Replace function:
$DateString = "2021-07-22"
$FormattedDate = $DateString.Replace('-', '/')
Usage and Examples for Each Function
Both the Split() function and the Replace function offer valuable functionality for manipulating strings in PowerShell. By understanding how to use these functions effectively, you can improve the efficiency and readability of your scripts. The following are examples of their usage:
# Splitting a string into an array of words
$Sentence = "This is a sample sentence."
$Words = $Sentence.Split(' ')
# Replacing all occurrences of a word in a string
$Text = "The quick brown dog jumped over the lazy dog."
$ModifiedText = $Text.Replace('dog', 'fox')
By combining these functions with the concatenation techniques discussed earlier, you can create powerful and flexible scripts that handle a wide range of string manipulation tasks in PowerShell.
Best Practices for Concatenating Strings in PowerShell
In this section, we will discuss best practices for concatenating strings in PowerShell, which will help you create efficient and readable scripts while avoiding common pitfalls and optimizing performance.
Tips for Efficient and Readable String Concatenation
When concatenating strings in PowerShell, it’s essential to prioritize readability and efficiency. Some tips to achieve this include:
- Choose the appropriate concatenation technique based on the specific use case and complexity of the strings.
- Use the format (-f) operator for complex string concatenation, as it improves readability and simplifies your script.
- When concatenating strings with variables, use double quotes to embed the variables directly in the string, making it more readable.
- Opt for the join operator when concatenating multiple strings, as it enhances readability and simplifies the code.
Avoiding Common Pitfalls
To ensure the success of your PowerShell script, it’s crucial to avoid common pitfalls when concatenating strings. Some common issues to watch out for include:
- Incorrectly concatenating strings and integers, which can lead to errors or unexpected results. Instead, use appropriate techniques such as typecasting or the -f operator to handle this scenario.
- Overusing the plus (+) operator, which can impact the script’s performance. Consider using alternative concatenation methods like the join operator or the format operator for better performance.
- Not considering the impact of special characters in your strings. Remember to handle escape characters properly to avoid issues.
Optimizing Performance While Concatenating Strings
Performance optimization is essential when working with PowerShell scripts, especially when dealing with large-scale operations or extensive string concatenation. Here are some tips to enhance the performance of your string concatenation:
- Use the StringBuilder class for large-scale concatenation operations, as it offers significant performance benefits compared to standard concatenation methods.
- Avoid using the plus (+) operator excessively, as it may lead to poor performance. Opt for alternative techniques like the join operator or the format operator, which offer better performance.
- When possible, use pipeline operations to process and concatenate strings, as they can improve performance by reducing memory usage and processing overhead.
By following these best practices, you can create efficient, readable, and high-performing PowerShell scripts that effectively handle string concatenation tasks.
Conclusion
In this article, we have discussed various best practices and techniques for concatenating strings in PowerShell. We have explored basic concatenation using the plus (+) operator, the join operator, the format (-f) operator, as well as more advanced methods such as the Concat() method and the StringBuilder class. These techniques enable you to efficiently manipulate strings in your PowerShell scripts, ensuring readability and optimal performance.
Mastering string concatenation is crucial for effective scripting in PowerShell. By understanding and implementing the best practices discussed here, you will be better equipped to write robust and efficient scripts that can handle complex operations and data manipulation tasks.
We encourage you to continue exploring the powerful features and functionalities of PowerShell, as it is an invaluable tool for Windows system administrators and developers alike. With a deeper understanding of PowerShell’s capabilities, you will be able to tackle a wide range of automation and scripting challenges, making your work more efficient and streamlined.
Remember to visit our website for more informative articles, guides, and troubleshooting tips related to Windows 10, Windows 11, and other technology topics. We aim to provide valuable insights and resources to help you make the most of your Windows experience.
Discover More Windows Tips
Mastering string concatenation in PowerShell can elevate your scripting skills, allowing you to create more efficient and powerful scripts. With this knowledge, you can confidently tackle more advanced topics related to Windows 10 and Windows 11. To dive deeper into Windows operating systems, we invite you to visit our website for comprehensive guides, troubleshooting tips, and more!